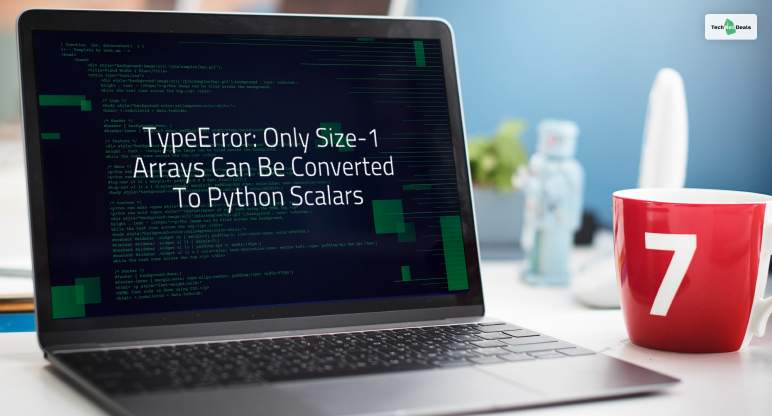
The error, “only size-1 arrays can be converted to Python scalars,” comes only when you made input of an invalid data type within a scalar value returning function. Using the solutions provided in this article, you can prevent the problem from happening and can successfully run your Python program.
“TypeError: Only Size-1 Arrays Can Be Converted To Python Scalars” – Major Causes
There are two significant causes through which the TypeError can occur. Both of these causes are due to the error from the side of the user and can be prevented easily with the use of appropriate parameters. The reasons are given below:
1. Making Use Of Incorrect Datatype
All data types in Python have their unique attributes and methods. All these datatypes are used differently in different cases. Most NumPy methods support only arrays of single value or size-1. If you use a NumPy array in these methods, you will surely get the TypeError.
Example:
import numpy as nmp x= nmp.array([1, 2, 3])x= nmp.int(x) Output: TypeError: only size-1 arrays can be converted to Python scalars |
Here, the array “x” contains only integer elements, and if you try to convert this array into type int, it will give you the TypeError, since nmp.int() only accepts single values.
2. Making Use Of Single Conversion Function
In the single conversion function, you can only provide datatypes that are single-valued and further convert those datatypes. An example of single-valued conversion is converting a string value into an int value. NumPy functions only accept the single NumPy element and, within it, change its datatype. Therefore, if you use NumPy array, you will surely get the TypeError.
Example:
import numpy as nmp x= nmp.array([1, 2, 3])x= nmp.float(x) Output: TypeError: only size-1 arrays can be converted to Python scalars |
Since this program is trying to convert every integer in the NumPy array to float-type, hence, it shows a TypeError at runtime.
Solutions For The Error “Only Size-1 Arrays Can Be Converted To Python Scalars.”
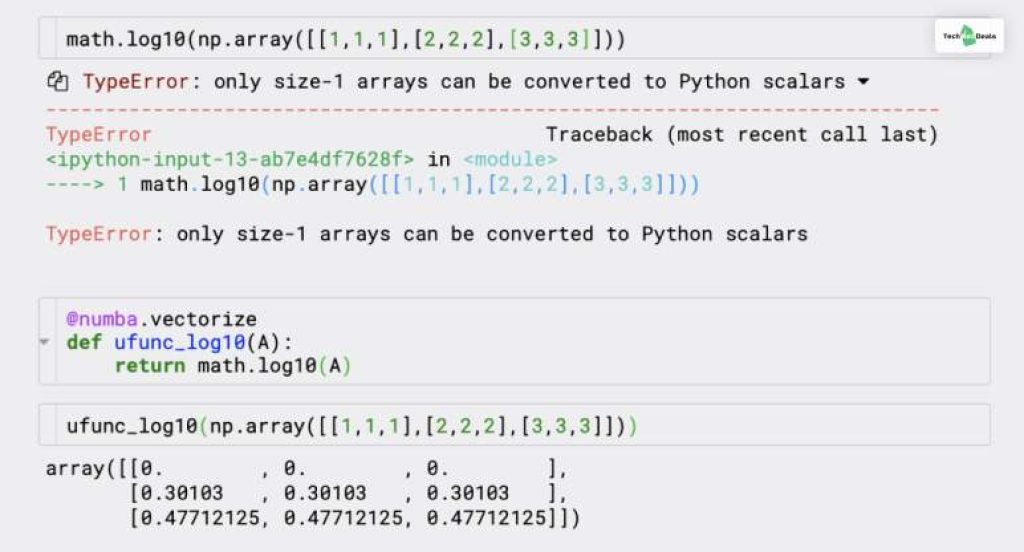
The following are the major resolutions which you can make use of to prevent the TypeError from returning “only size-1 arrays can be converted to Python scalars”:
Solution 1: Make Use Of The Vectorize Function From NumPy
The vectorize() function is used to apply the “vectorize” algorithm to the set of values within your program, apart from applying the algorithm to each value. Since the algorithm gets used on the sets of values, it leads to the TypeError: only size-1 arrays can be converted to Python scalars. By using the numpy.vectorize() function between the methods and the algorithm, you are making it act like the map() function without using it.
Example:
import numpy as nmp vec= nmp.vectorize(nmp.float)x= nmp.array([1, 2, 3])x= vec(x) print(x) Output: [1. 2. 3] |
Here, you have created a vector which is accepting nmp.float as a type of parameter. To apply your method on every NumPy array element, you need to use the vectorize() function. Then, you have created a NumPy array and used the vector “vec()” to apply nmp.float upon all your values. This method removes not only the TypeError problem but also converts every value into float-type.
Solution 2: Use The Map() Function Of NumPy
To solve the “TypeError: only size-1 arrays can be converted to Python scalars” error, you can also use an inbuilt Python function called map() over all your array elements in the program. Here, the map() function accepts two parameters:
- The function that you are applying over your sets of values.
- The array that you are looking to change in the program.
Example:
import numpy as nmp x= nmp.array([1, 2, 3])x= nmp.array(list(map(nmp.float, x))) print(x) Output: [1. 2. 3] |
In this case, you have initially created an integer array. Then you are using the function map (nmp.float, x) to convert every element starting from the NumPy array to a float integer. Since the map() function outputs a map object, you must try to convert it back to restore its datatype to its original list and NumPy array. In the end, you will not see any TypeError.
Solution 3: Make Use Of Loops In Your Code
With the help of loops, you are basically forcefully applying your function to a set of values. However, by making use of loops, you can get better control of every part of your elements. You can also use loops to customize your elements as per your needs.
Example:
import numpy as nmp x= nmp.array([1, 2, 3])y= nmp.array([None]*3) for i in range(3):y[i] = nmp.float(x[i]) print(y) Output: [1.0 2.0 3.0] |
Here, you are using the indexing process to get the primary integer in the program from your NumPy array. You are then applying nmp.float() method to convert your integer from float type to int type. Apart from that, here, you are also creating a dummy “y” NumPy array in your code, where you are storing your float values after it changes.
Solution 4: Use The “apply_along_axis” Method
With the help of the apply_along_axis() method, you can apply your function along a particular axis over the NumPy array. Here, the NumPy gets looped as per the number of the axis, and you can make use of it to set your values for applying your function in the code.
Example:
import numpy as nmp x= nmp.array([1, 2, 3])appl= lambda y: [nmp.float(i) for i in y] x= nmp.apply_along_axis(appl, 0, x)print (x) Output: [1. 2. 3.] |
Here, you are basically using the lambda function to create a vectorized function of the main function. Then you are using nmp.apply_along_axis for the application of the lambda function upon the particular NumPy array. Furthermore, you can also add the axis number on which you want to apply this function.
Summing Up
In this article, we have first explained what type of error is “only size-1 arrays can be converted to Python scalars” and what are the significant causes behind it. Furthermore, you have come across the major ways/solutions through the help of which you can prevent the error from happening.
However, if you have found a better solution than the ones provided above, then consider writing it down in the comments section below.
Read Also: